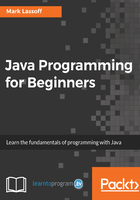
Using the pow() function
One of these functions should stick out for us, which is pow(), or the power function. It returns the value of the first argument (double a) raised to the power of the second argument (double b). In short, it will allow us to raise numbers to an arbitrary power:

Let's get back to coding. Alright, let's employ this pow() function to modify the value of our variable number after we've declared it. We're going to do something along the lines of number = pow, but we need a little more information:

How exactly do we employ this pow() function? Well, if we click on our documentation, we'll see that when the pow() function is declared, in addition to its name, there's also, between parentheses, two arguments specified. These arguments, double a and double b, are the two pieces of information the function is requesting before it can operate as expected.
Our job, in order to use this function, is to replace the requests double a and double b with actual variables or explicit values so that the pow() function can do its thing. Our documentation tells us that double a should be replaced with the variable or value that we'd like to raise to the power of double b.
So let's replace the first type argument with our variable number, which is what we want to raise to an arbitrary power. On that note, number is float not double, and that's going to give us some trouble unless we simply change it to double. So let's do that. For the second argument, we don't have a precreated variable to replace double b with, so let's just use an explicit value, such as 4.0:

Notice that I get rid of the double specifier when I call the pow() function. This specifier only exists to let us know what type Java is expecting.
In theory, the pow() function now has all the information it needs to go ahead and run and raise the value of our number variable to the power of 4. However, NetBeans is still giving us our red warning sign. Right now, that's because NetBeans, and Java by extension, doesn't know where to find this pow keyword. For the same reasons that we need to specify a full path to System.out.println(), we need to specify a full path in which it can find the pow() function for Java. This is the same path we followed to get to the pow() function in our documentation. So let's specify java.lang.Math.pow() as it's path in our code:
package themathlib; public class TheMathLib { public static void main(String[] args) { double number = 4.321; number = java.lang.Math.pow(number, 4.0); System.out.println(number); } }
Now we're pretty much good to go. Let's utilize the number variable once in our println statement, then we should be able to run our program:

We can plug it into our calculator if we want, but I'm pretty confident that our program has outputted the value of 4.321 raised to the power of 4.
So this is great! We've just employed external code to not only make our program easier to write, but also to keep it very human-readable. It required much fewer lines of code than it would have otherwise.