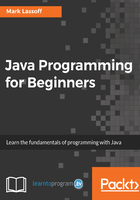
The Math class library
A good portion of our time on any software development project is going to be spent teaching our program to solve the types of problems it would comes across on a regular basis. As programmers, we too will run into certain problems time and time again. Sometimes, we'll need to code our own solutions to these problems and hopefully save them for later use. However, more often than not, someone has run into these problems before, and if they've made their solution publicly available, one of our options is to leverage their solution for our own gain.
In this section, we'll use the Math class library, which is bundled with the JDK to solve some math problems for us. To start this section, create a brand new NetBeans project (I'm going to name it TheMathLib) and enter the main() function. We're going to write a very simple program. Let's declare a floating-point number variable and give it a value (don't forget the f alphabet at the end of our explicit number to let Java know that we've declared a floating-point number), then use System.out.println() to print this value to the screen:
package themathlib; public class TheMathLib { public static void main(String[] args) { float number = 4.321f; System.out.println(number); } }
OK, there we go:

Now, with this program, we'd like to make it really easy to raise our floating-point number to various powers. So, if we simply want to square this number, I guess we could just print out the value of number*number. If we want to cube it, we could print out number*number*number. And, if we want to raise it to the power of six, we could multiply it six times by itself. Of course, this gets unwieldy pretty quickly, and there's certainly a better way.
Let's leverage the Java Math class library to help us raise numbers to varying exponential powers. Now, I've just told you that the functionality we're looking for lives in the Math class library. This is the kind of push in the right direction you should expect to get from a Google search, or if you're an experienced software developer, you can implement a specific API. Unfortunately, that's not quite enough information for us to start using the functionality of this class library. We don't know the specifics of how it works or even exactly what functionality it offers us.
To find this out, we're going to have to look at its documentation. Here's the documentation web page managed by Oracle for the libraries found in our Java Development Kit: docs.oracle.com/javase/7/docs/api/. Among the libraries that show up on the page is java.lang. When we select it, we'll find the Math class that we've been looking for under Class Summary. Once we navigate to the Math class library page, we get two things. First we get some human-friendly text write-up about the library, its history, what its intended uses are, very meta-level stuff. If we scroll down, we see the functionality and methods implemented by the library. This is the nitty-gritty of where we'd like to be:
