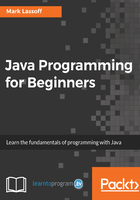
The double data type
Let's quickly talk about the double data type. It is a sister type of float. It provides a greater resolution: double numbers can have even more decimal places. But they take up a little more memory. At this point in time, using double or float is almost always a style or personal preference decision. Unless you're working with complicated software that must run at peak memory efficiency, the extra memory space taken up by a double is not very significant.
To illustrate how double works, let's change the two integer numbers in our FloatingPointNumbers.java program to a double data type. When we only change the names of the variables, the logic of our program doesn't change at all. But when we change the declaration of these variables from declaring integers to doubles, the logic does change. Anyway, when we explicitly declare a number with decimal places, it defaults to being double:

Now we need to fix the error. The error occurs because dividing a double data type by another one is going to return a double result. We can solve this issue in two ways:
- First, we could cast dNumber1 and dNumber2 to floating-point numbers and then divide them:
float fNumber = (float) dNumber1/ (float) dNumber2;
- However, dividing our two double numbers by each other is a perfectly legitimate operation. So why not allow this to occur naturally and then cast the resulting double to a floating-point number, thereby preserving a greater amount of resolution. Just like in algebra, we can break up conceptual blocks of our program that we would like to occur before another block using parentheses:
float fNumber = (float) (dNumber1/dNumber2);
Now if we run this program, we get the expected result:
