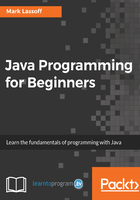
Importing class libraries
One thing that's not super human-readable about our program is the long paths to functions such as pow() and println(). Is there a way we could shorten them? There certainly is. If the makers of Java had wanted to, they could have allowed us to call this function by simply typing Math.pow() in all instances. This unfortunately would have some unintended side effects. For example, if there were two libraries linked up to Java and they both declared a Math.pow() function, Java would not know which one to use. Hence, by default, we're expected to link to libraries directly and explicitly.
So, if we'd like to just be able to type out something like Math.pow(), we can import a library into the local space that we're working in. We just need to do an import command above our class and the main() function declaration. All the import command takes as input is the path that we'd like Java to look for when it comes across a keyword, such as pow(), that it doesn't immediately recognize. In order to allow us to employ the easier syntax Math.pow() in our program, we simply need to type import java.lang.Math:
package themathlib; import java.lang.Math; public class TheMathLib { public static void main(String[] args) { double number = 4.321; number = java.lang.Math.pow(number, 4.0); System.out.println(number); } }
There is some special syntax for imports. Let's say we wanted to import all the class libraries in java.lang. To do this, we could replace .Math with .* and make it java.lang.* which translates to "import every library from the java.lang package." I should probably inform you that for those of us working in NetBeans, this import is done by default. However, in this case, we're going to do it explicitly because you may have to do this while working in other Java environments as well.