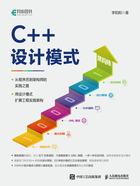
上QQ阅读APP看书,第一时间看更新
1.3.2 程序应用
EIT造型迁移到编程实践中,E是基类、I是接口、T是派生类,应用到1.1节的Car类的案例中,Car是E、SetDiffTire(string tire)是I、DZ是T,用UML类图表示的EIT造型如图1-10所示。
图1-10说明了EIT造型的程序应用,基类Car是EIT造型中的E,基类中的虚方法SetDiffTire (string tire)是EIT造型中的I,派生类DZ是EIT造型中的T,派生类实现具体的SetDiffTire(string tire)(接口I)。

▲图1-10 EIT造型的程序应用
图1-10中,Car与DZ紧耦合,在软件设计中可以继续优化,将Car与DZ分离(解耦),增加一个接口类SetTireInterface,在Car类中实现安装不同轮胎的方法DiffTire();各个派生类继承接口类,例如,DZInterface派生类继承自接口类SetTireInterface,DZInterface派生类“安装”符合自身应用需求的Tire,具体代码如下。
//新增接口类 class SetTireInterface { public: SetTireInterface(string tn):m_tireName(tn){} virtual string SetDiffTire() = 0; protected: string m_tireName; }; //新增接口派生类 class DZInterface:public SetTireInterface { public: DZInterface(string tn):SetTireInterface (tn){} string SetDiffTire() { return m_tireName; } }; //Car与DZ解耦 class Car { public: Car(string en):engineName(en) { m_Interface = new DZInterface("miqilin"); } void SetCommonEngine() { cout<<"commonEngine is: "<< engineName<<endl; } void DiffTire() { cout<<m_Interface->SetDiffTire()<<endl; } protected: string engineName; SetTireInterface* m_Interface; }; //客户端主程序 int main() { Car *car = new Car("weichai"); car->SetCommonEngine(); car->DiffTire(); delete car; }