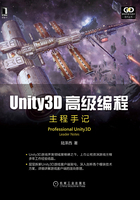
上QQ阅读APP看书,第一时间看更新
4.4.2 Culling模块
Culling是对模型进行裁剪的工具类,大都用在Mask(遮罩)上,只有Mask才有裁剪的需求。
如图4-4所示,文件夹中包含四个文件,其中一个是静态类,一个是接口类。

图4-4 Culling模块的文件夹结构
Clipping类中有两个函数比较重要,常被用在Mask的裁剪上,其源代码如下:
public static Rect FindCullAndClipWorldRect(List<RectMask2D>rectMaskParents, out bool validRect) { if (rectMaskParents.Count == 0) { validRect = false; return new Rect(); } var compoundRect = rectMaskParents[0].canvasRect; for (var i = 0; i<rectMaskParents.Count; ++i) compoundRect = RectIntersect(compoundRect, rectMaskParents[i].canvasRect); var cull = compoundRect.width<= 0 || compoundRect.height<= 0; if (cull) { validRect = false; return new Rect(); } Vector3 point1 = new Vector3(compoundRect.x, compoundRect.y, 0.0f); Vector3 point2 = new Vector3(compoundRect.x + compoundRect.width, compoundRect.y + compoundRect.height, 0.0f); validRect = true; return new Rect(point1.x, point1.y, point2.x - point1.x, point2.y - point1.y); } private static Rect RectIntersect(Rect a, Rect b) { float xMin = Mathf.Max(a.x, b.x); float xMax = Mathf.Min(a.x + a.width, b.x + b.width); float yMin = Mathf.Max(a.y, b.y); float yMax = Mathf.Min(a.y + a.height, b.y + b.height); if (xMax>= xMin && yMax>= yMin) return new Rect(xMin, yMin, xMax - xMin, yMax - yMin); return new Rect(0f, 0f, 0f, 0f); }
上述代码中的函数为Clipping类里的函数,第一个函数FindCullAndClipWorldRect()的含义是计算RectMask2D重叠部分的区域。第二个函数RectIntersect()为第一个函数提供计算服务,其含义是计算两个矩阵的重叠部分。
这两个函数都是静态函数,也可视为工具函数,直接调用即可,不需要实例化。