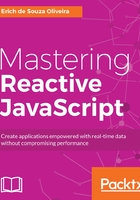
Observable node event emitter (fromEvent)
Lots of Node.js modules implement an event emitter, which is basically an object with the on() method that is called every time an event happens. We can transform these objects into EventStreams of bacon.js to take advantage of all the transformations. The method fromEvent() has the following signature:
Bacon
.fromEvent(eventEmitter,eventName);
The parameters are eventEmitter; the node eventEmitter object; and eventName, the name of the event. We can use the fs module to show the usage of this method.
To read a file using the fs node module that uses an event emitter, we need to create a readStream for the file using the following code:
var fs = require('fs');
var FILE_PATH = 'SOME FILE';
var readStream = fs.createReadStream(FILE_PATH,'utf8');
Then, subscribe to the events on this emitter using the on() method, as follows:
readStream.on('data',(content)=>console.log(content));
To transform this code into a code using a bacon.js EventStream, we need to change the last line of the following code:
var eventStream = Bacon.fromEvent(readStream,'data');
eventStream
.onValue((content)=> console.log(content));
So comparing with the method signature, our eventEmitter parameter is the readStream object and eventName is data.
The fromEvent() method can also be used to listen to DOM events (similar to the asEventStream() method), as you can see in the following code:
var clickEventStream = Bacon.fromEvent(document.getElementById('#myButton'), 'click');