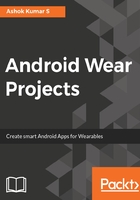
Creating the packages
Creating packages helps in maintaining the code in a structured manner. In the Wear-note-1 application, we will create the following package structure:
- model
- utils
- adapter
- activity
Create these packages in the Wear application and add the activity inside the activity package. The following screenshot explains how the package looks:

- All the packages inside the Wear project scope.
- Main Activity moved inside the activity package.
The conceptualized Wear note-taking app should save notes and should be able to delete notes. For persisting the notes, we shall use SharedPreferences, which allows all the Create, Read, Update, and Delete (CRUD) operations in terms of key-value pairs. SharedPreferences wraps inside the Android SDK; we need not worry about installing a third-party library for this chapter.
Let's create a POJO model called Note.java inside the model package in the Wear project scope. And let's create the following member variables inside the Note.java class:
private String notes = ""; private String id = "";
After creating the previous member variables, we shall create a parameterized constructor as follows:
public Note(String id, String notes) { this.id = id; this.notes = notes; }
Once the constructor is completed, we need to create setters and getters for the same member variables. Android Studio helps in creating setters and getters for the requested data type using the following shortcut:

Use the previous shortcut in the Generate... option and select Getter and Setter. Then, choose the variables that need Getter and Setter. For this note application, we only have the id and actual note. We shall choose id and note.
The complete Note.java class looks as follows:
package com.ashok.packt.wear_note_1.model; /** * Created by ashok.kumar on 15/02/17. */ public class Note { private String notes = ""; private String id = ""; public Note(String id, String notes) { this.id = id; this.notes = notes; } public String getNotes() { return notes; } public void setNotes(String notes) { this.notes = notes; } public String getId() { return id; } public void setId(String id) { this.id = id; } }
Now, this POJO class will take care of setting and getting the value from and to SharedPreference.