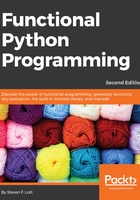
Pure functions
To be expressive, a function should be free from the confusion created by side effects. Using pure functions can also allow some optimizations by changing evaluation order. The big win, however, stems from pure functions being conceptually simpler and much easier to test.
To write a pure function in Python, we have to write local-only code. This means we have to avoid the global statements. We need to look closely at any use of nonlocal; while it is a side effect in some ways, it's confined to a nested function definition. This is an easy standard to meet. Pure functions are a common feature of Python programs.
There isn't a trivial way to guarantee a Python function is free from side effects. It is easy to carelessly break the pure function rule. If we ever want to worry about our ability to follow this rule, we could write a function that uses the dis module to scan a given function's __code__.co_code compiled code for global references. It could report on use of internal closures, and the __code__.co_freevarstuple method as well. This is a rather complex solution to a rare problem; we won't pursue it further.
A Python lambda is a pure function. While this isn't a highly recommended style, it's always possible to create pure functions through the lambda objects.
Here's a function created by assigning lambda to a variable:
>>> mersenne = lambda x: 2**x-1 >>> mersenne(17) 131071
We created a pure function using lambda and assigned this to the variable mersenne. This is a callable object with a single parameter, x, that returns a single value. Because lambdas can't have assignment statements, they're always pure functions and suitable for functional programming.