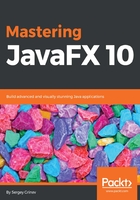
上QQ阅读APP看书,第一时间看更新
Application class
The most common way to use the JavaFX API is to subclass your application from the javafx.application.Application class. There are three overridable methods in there:
- public void init(): Overriding this method allows you to run code before the window is created. Usually, this method is used for loading resources, handling command-line parameters, and validating environments. If something is wrong at this stage, you can exit the program with a friendly command-line message without wasting resources on the window's creation.
Note this method is not called on JavaFX Application Thread, so you shouldn't construct any objects that are sensitive to it, such as Stage or Scene.
- public abstract void start(Stage stage): This is the main entry point and the only method that is abstract and has to be overridden. The first window of the application has been already prepared and is passed as a parameter.
- public void stop(): This is the last user code called before the application exits. You can free external resources here, update logs, or save the application state.
The following JavaFX code sample shows the workflow for all these methods:
Note the comment in the first line—it shows the relative location of this code sample in our book's GitHub repository. The same comment will accompany all future code samples, for your convenience.
// chapter1/HelloFX.java
import javafx.application.Application;
import javafx.scene.*;
import javafx.stage.Stage;
public class FXApplication extends Application {
@Override
public void init() {
System.out.println("Before");
}
@Override
public void start(Stage stage) {
Scene scene = new Scene(new Group(), 300, 250);
stage.setTitle("Hello World!");
stage.setScene(scene);
stage.show();
}
public void stop() {
System.out.println("After");
}
}
Note that you don't need the main() method to run JavaFX. For example, this code can be compiled and run from the command line:
> javac FXApplication.java
> java FXApplication
It shows a small empty window:
