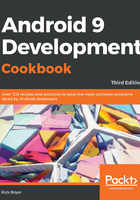
上QQ阅读APP看书,第一时间看更新
How to do it...
Since this recipe is building on the previous recipe, most of the work is already done. We'll add an EditText element to the main activity so that we have something to send to SecondActivity. We'll use the (auto-generated) TextView view to display the message. The following are the complete steps:
- Open activity_main.xml and add the following <EditText> element above the button:
<EditText
android:id="@+id/editTextData"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toTopOf="@+id/button" />
The <Button> element that we created in the previous recipe doesn't change.
- Now, open the MainActivity.java file and change the onClickSwitchActivity() method as follows:
public void onClickSwitchActivity(View view) { EditText editText = (EditText)findViewById(R.id.editTextData); String text = editText.getText().toString(); Intent intent = new Intent(this, SecondActivity.class); intent.putExtra(Intent.EXTRA_TEXT,text); startActivity(intent); }
- Next, open the activity_second.xml file and add the following <TextView> element:
<TextView
android:id="@+id/textViewText"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toTopOf="@id/buttonClose"/>
- The last change is to edit the second activity to look for this new data and display it on the screen. Open SecondActivity.java and edit onCreate() as follows:
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_second);
TextView textView = (TextView) findViewById(R.id.textViewText);
if (getIntent() != null && getIntent().hasExtra(Intent.EXTRA_TEXT)) {
textView.setText(getIntent().getStringExtra(Intent.EXTRA_TEXT));
}
}
- Now, run the project. Type some text in the main activity and press Launch Second Activity to see it send the data.