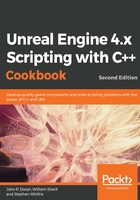
上QQ阅读APP看书,第一时间看更新
How to do it...
To create your own UObject derivative class, follow these steps:
- From your running project, select File | New C++ Class inside the UE4 editor.
- In the Add C++ Class dialog that appears, go to the upper-right-hand side of the window and tick the Show All Classes checkbox:

- Select Object (top of the hierarchy) as the parent class to inherit from, and then click on Next.
Note that although Object will be written in the dialog box, in your C++ code, the C++ class you will be deriving from is actually UObject with a leading uppercase U. This is the naming convention of UE4.
UCLASS deriving from UObject (on a branch other than Actor) must be named with a leading U.
UCLASS deriving from Actor must be named with a leading A ( Chapter 4, Actors and Components).
C++ classes (that are not UCLASS) deriving from nothing do not have a naming convention, but can be named with a leading F (for example, FAssetData), if preferred.
Direct derivatives of UObject will not be level-placeable, even if they contain visual representation elements such as UStaticMeshes. If you want to place your object inside a UE4 level, you must at least derive from the Actor class or beneath it in the inheritance hierarchy. See Chapter 4, Actors and Components, for more information how to derive from the Actor class for a level-placeable object.
UCLASS deriving from UObject (on a branch other than Actor) must be named with a leading U.
UCLASS deriving from Actor must be named with a leading A ( Chapter 4, Actors and Components).
C++ classes (that are not UCLASS) deriving from nothing do not have a naming convention, but can be named with a leading F (for example, FAssetData), if preferred.
Direct derivatives of UObject will not be level-placeable, even if they contain visual representation elements such as UStaticMeshes. If you want to place your object inside a UE4 level, you must at least derive from the Actor class or beneath it in the inheritance hierarchy. See Chapter 4, Actors and Components, for more information how to derive from the Actor class for a level-placeable object.
This chapter's example code will not be placeable in the level, but you can create and use blueprints based on the C++ classes that we write in this chapter in the UE4 editor.
- Name your new Object derivative something appropriate for the object type that you are creating. I'll call mine UserProfile:

This comes off as UUserObject in the naming of the class in the C++ file that UE4 generates to ensure that the UE4 conventions are followed (In C++, class names with a UCLASS are preceded with a leading U).
- Click on Create Class and the files should be created after file compilation is completed. Afterwards, Visual Studio should open (otherwise, open the solution by going to File | Open Visual Studio), and will open up the .cpp file of the class we just created (UserProfile.cpp). Open the header rule (UserProfile.h) for your class and ensure your class file has the following form:
#pragma once
#include "CoreMinimal.h"
#include "UObject/NoExportTypes.h"
#include "UserProfile.generated.h"
/**
*
*/
UCLASS()
class CHAPTER_02_API UUserProfile : public UObject
{
GENERATED_BODY()
};
- Compile and run your project. You can now use your custom UCLASS object inside Visual Studio, as well as inside the UE4 editor. See the following recipes for more details on what you can do with it.
How to create and destroy your UObject -derived classes is outlined in the Instantiating UObject-derived classes (ConstructObject <> and NewObject <>) and Destroying UObject-derived classes recipes later in this chapter.