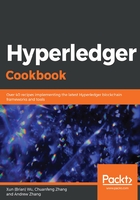
上QQ阅读APP看书,第一时间看更新
Writing Node.js sever-side code
We have now set up the client environment. We should be able to connect to the remote Fabric network and trigger the chaincode API. In our Node.js app, create the app.js file. This file will act as a connector to create a new gateway to connect to our peer node. It also creates a new filesystem-based wallet for managing identities. Once we have connected to the peer node, the function in app.js can find a contract through the channel and then submit the specified transaction. To do this, follow these steps:
- Create the wallet file:
async function ship() {
try {
// Create a new file system based wallet for managing identities.
const walletPath = path.join(process.cwd(), 'wallet');
const wallet = new FileSystemWallet(walletPath);
..
}
- The wallet file needs to make sure the user exists by using the wallet.exist API:
const userExists = await wallet.exists('user1');
if (!userExists) {
console.log('An identity for the user "user1" does not exist in the wallet');
console.log('Run the registerUser.js application before retrying');
return;
}
- Connect to blockchain through gateway, bypassing the wallet and identity information. Once connected to the network successfully, get the assetmgr contract from the network:
// Create a new gateway for connecting to our peer node.
const gateway = new Gateway();
await gateway.connect(ccp, { wallet, identity: 'user1', discovery: { enabled: false } });
// Get the network (channel) our contract is deployed to.
const network = await gateway.getNetwork('mychannel');
// Get the contract from the network.
const contract = network.getContract('assetmgr');
- Submit a transaction to invoke the chaincode function:
// Submit the specified transaction.
await contract.submitTransaction("Ship", "100", "OEM deliver ipad to school", "New Jersey");
- Disconnect from gateway and return to the client caller. To do this, follow the code:
// Disconnect from the gateway.
await gateway.disconnect();
With node.js, you need set up your node.js server listen, of course.
app.listen(3000, function () {
console.log('Example app listening on port 3000!');
});