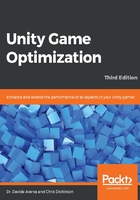
Message cleanup
Since message objects are classes, they will be created dynamically in memory and will be disposed of shortly afterward when the message has been processed and distributed among all listeners. However, as you will learn in Chapter 8, Masterful Memory Management, this will eventually result in garbage collection, as memory accumulates over time. If our application runs for long enough, it will eventually result in occasional garbage collection, which is the most common cause of unexpected and sudden CPU performance spikes in Unity applications. Therefore, it is wise to use the messaging system sparingly and avoid spamming messages too frequently on every update.
The more important cleanup operation to consider is the deregistration of delegates if an object needs to be destroyed. If we don't handle this properly, then the messaging system will hang on to delegate references that prevent objects from being fully destroyed and freed from memory.
Essentially, we will need to pair every AttachListener() call with an appropriate DetachListener() call when the object is destroyed or disabled or we otherwise decide that we no longer need it to be queried when messages are being sent.
The following method definition in the MessagingSystem class will detach a listener for a specific event:
public bool DetachListener(System.Type type, MessageHandlerDelegate handler) {
if (type == null) {
Debug.Log("MessagingSystem: DetachListener failed due to having no " +
"message type specified");
return false;
}
string msgType = type.Name;
if (!_listenerDict.ContainsKey(type.Name)) {
return false;
}
List<MessageHandlerDelegate> listenerList = _listenerDict[msgType];
if (!listenerList.Contains (handler)) {
return false;
}
listenerList.Remove(handler);
return true;
}
Here is an example usage of the DetachListener() method added to our EnemyManagerWithMessagesComponent class:
void OnDestroy() {
if (MessagingSystem.IsAlive) {
MessagingSystem.Instance.DetachListener(typeof(EnemyCreatedMessage),
this.HandleCreateEnemy);
}
}
Note how this definition makes use of the IsAlive property declared in the SingletonComponent class. This safeguards us against the aforementioned problem of accidentally creating a new MessagingSystem class during application shutdown since we can never guarantee that the singleton gets destroyed last.